Internationalization
ZingGrid has smart defaults for rendering <zg-column>
with type number
, date
, and currency
through internationalization. Implementing this feature will enable smart filtering, sorting, searching, and the dir
attribute.
Detecting Language/ Locale
English is the default language used for grids, even if <html lang="en">
is not defined. When you define another language, it will look for that registered language file. For example, if you define [lang="es"]
, the grid will look for the es.js
file.
If that file doesn't exist, then the grid will use smart defaults (algorithm defined below) for that language as best as it can for currency and date columns.
Algorithm
ZingGrid goes through several layers to detect the language/locale for the page. In this order, ZingGrid checks:
- The language set on the grid itself (e.g.,
<zing-grid lang="es">
) - The language set via
registerLanguage
with the default set to true (e.g.,ZingGrid.registerLanguage(newLang, ‘en-US-x-twain’, true)
) - The language set via
setLanguage
(e.g.,ZingGrid.setLanguage(‘es’)
) - The language set on the HTML tag (e.g.,
<html lang="de">
) - English
At each layer, we will start with the locale and break it down all the way to the language. If we ever get a match with built-in or a user added language file, we stop there.
For example, if lang
is set to en-US-x-pirate:
- We will first look for a language match for en-US-x-pirate
- If there is no match, we will then look at en-US-x
- If there is no match, we will look at en-US
- If there is no match, we will look at en
Setting Language/ Locale
Columns with type
currency, locale, and number will take a lang
and locale
attribute to properly display the given data. If these attributes are not provided, ZingGrid will look at the hierarchy specified above and find the most appropriate language and locale.
tag
You can set the language globally on the <html>
tag with the lang
attribute, like so:
<html lang="es">...</html>
tag
You can set the language per grid on the <zing-grid>
tag with the lang
attribute, like so:
<zing-grid lang="es"></zing-grid>
ZingGrid.setLanguage()
You can set the language globally with the API method setLanguage()
, like so:
ZingGrid.setLanguage('es');
Custom Language
You can set a custom language by registering a language file with ZingGrid.registerLanguage(obj, 'custom')
and the appropriate JavaScript object.
lang file
You will need to define a language file to create a custom mapping using our default export object (see below). You will typically name this language file the same as the language you are creating. For example, if [lang="es"]
, then you will create a file called es.js
.
You can view the full default en.js
language file online on our GitHub. We recommend using this as a reference to starting your own custom language.
export default { lang: 'en', columntypes: { editor: 'Edit', remover: 'Delete', selector: 'Select', }, context: { copyCell: 'Copy Cell', pasteCell: 'Paste Cell', insertRecordLabel: 'Insert New Record', insertRecordHere: 'Here', insertRecordEnd: 'End', insertRecordStart: 'Start', deleteRow: 'Delete row', sortColumn: 'Sort Column', editCell: 'Edit Cell', editRow: 'Edit Row', selectCell: 'Select Cell', selectRow: 'Select Row', deselect: 'Deselect', previousPage: 'Go to previous page', nextPage: 'Go to next page', firstPage: 'Go to first page', lastPage: 'Go to last page', version: 'ZingGrid Version', save: 'Save', cancel: 'Cancel', }, date: { shortMonth: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'], longMonth: ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'], twoWeek: ['Su', 'Mo', 'Tu', 'We', 'Th', 'Fr', 'Sa'], shortWeek: ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'], longWeek: ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'], am: 'am', pm: 'pm', startWeek: 0, startYear: 6, } ... };
ZingGrid.registerLanguage()
Once you have defined a custom language file, you will use ZingGrid.registerLanguage(obj, 'custom')
to actually register your custom language to the page (see below). There is typically a direct one-to-one mapping between your export lang
key.
The second parameter of ZingGrid.registerLanguage
can be used to register the language as a different name than your export.
let myLang = { lang: 'custom', context: { previousPage: 'custom-Go to previous page', nextPage: 'custom-Go to next page', firstPage: 'custom-Go to first page', lastPage: 'custom-Go to last page' } ... }; ZingGrid.registerLanguage(myLang, 'custom');
Custom Language Grid
Here is a complete grid with a custom language defined:
Column Types
Internationalization affects default column behavior. For example, if you define <zg-column type="date" locale="de">
, it will add the appropriate date formatting to that column. The columns will default to the global <html lang="">
attribute, then <zing-grid lang="">
, and then <zg-column lang="">
will override them all because it is the most specific.
The following column types have special language default formatting:
<zg-column type="currency" type-currency="EUR">
<zg-column type="date">
<zg-column type="number">
If you want your data output in a different format, you can use a renderer or leave the column type as text and have it display exactly as the data is provided.
Currency
Your currency thousands separator will automatically format to the corresponding language default.
You still need the [type-currency="EUR"]
attribute to define the currency symbol.
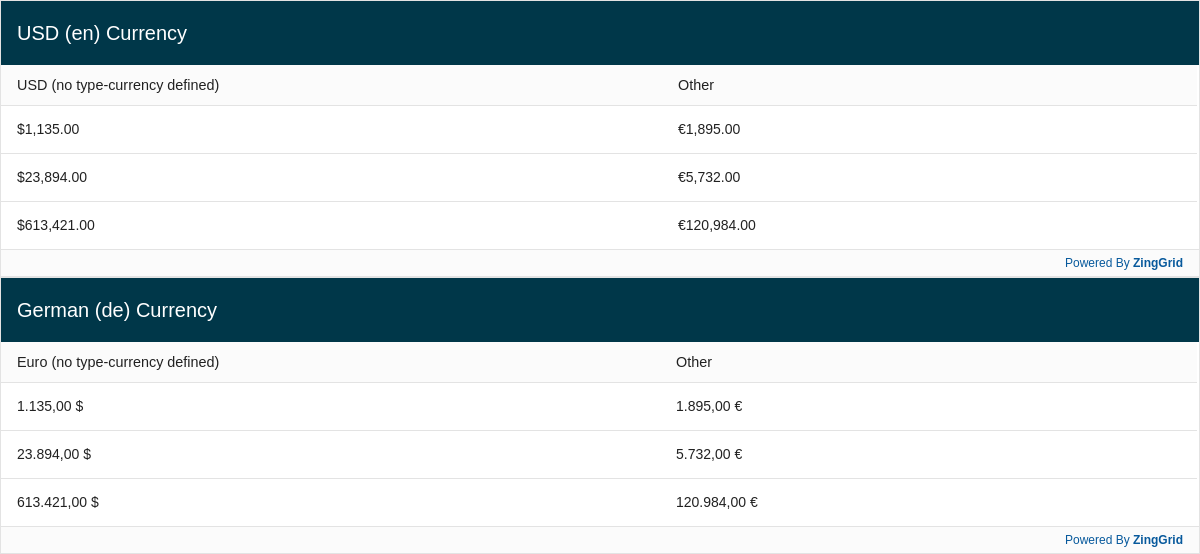
Number
Your number thousands separator will automatically format to the corresponding language default.
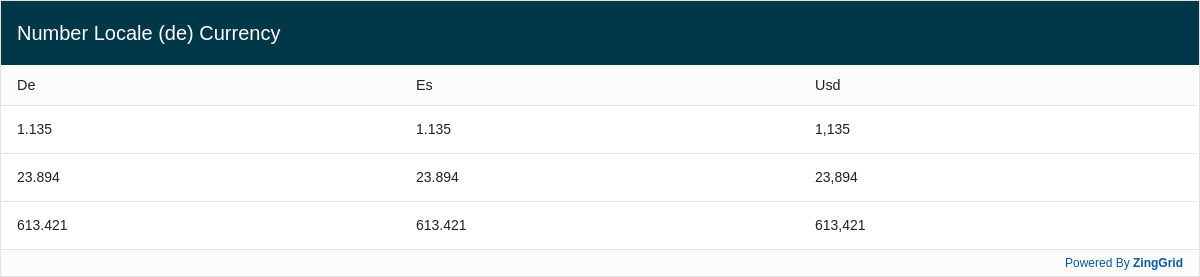
Date
Your date will automatically format to the corresponding language default. Add conventionally standard tokens (standard derived from moment.js) to date columns for custom date formatting, like so:
<zg-column type="date" type-date-format="[Month:] MM">
Date Tokens
Below is a comprehensive list of defined tokens in ZingGrid for date formatting with the attribute type-date-format
.
Any tokens here that refer to a string (MMMM, dddd, etc.) must be set via a language file. It will take the strings from the language file being used for the specific column or the grid.
Grouping | Token | Output |
---|---|---|
Month | M | 1 2 ... 11 12 |
Mo | 1st 2nd ... 11th 12th | |
MM | 01 02 ... 11 12 | |
MMM | Jan Feb ... Nov Dec | |
MMMM | January February ... November December | |
Quarter | Q | 1 2 3 4 |
Qo | 1st 2nd 3rd 4th | |
Day of Month | D | 1 2 ... 30 31 |
Do | 1st 2nd ... 30th 31st | |
DD | 01 02 ... 30 31 | |
Day of Year | DDD | 1 2 ... 364 365 |
DDDo | 1st 2nd ... 364th 365th | |
DDDD | 001 002 ... 364 365 | |
Day of Week | d | 0 1 ... 5 6 |
do | 0th 1st ... 5th 6th | |
dd | Su Mo ... Fr Sa | |
ddd | Sun Mon ... Fri Sat | |
dddd | Sunday Monday ... Friday Saturday | |
Day of Week (Locale) | e | 0 1 ... 5 6 |
Day of Week (ISO) | E | 1 2 ... 6 7 |
Week of Year | w | 1 2 ... 52 53 |
wo | 1st 2nd ... 52nd 53rd | |
ww | 01 02 ... 52 53 | |
Week of Year (ISO) | W | 1 2 ... 52 53 |
Wo | 1st 2nd ... 52nd 53rd | |
WW | 01 02 ... 52 53 | |
Year | YY | 70 71 ... 29 30 |
YYYY | 1970 1971 ... 2029 2030 | |
Y | 1970 1971 ... 9999 +10000 +10001 Note: This complies with the ISO 8601 standard for dates past the year 9999 | |
Week Year | gg | 70 71 ... 29 30 |
gggg | 1970 1971 ... 2029 2030 | |
Week Year (ISO) | GG | 70 71 ... 29 30 |
GGGG | 1970 1971 ... 2029 2030 | |
AM/PM | A | AM PM |
a | am pm | |
Hour | H | 0 1 ... 22 23 |
HH | 00 01 ... 22 23 | |
h | 1 2 ... 11 12 | |
hh | 01 02 ... 11 12 | |
k | 1 2 ... 23 24 | |
kk | 01 02 ... 23 24 | |
Minute | m | 0 1 ... 58 59 |
mm | 00 01 ... 58 59 | |
Second | s | 0 1 ... 58 59 |
ss | 00 01 ... 58 59 | |
Fractional Second | S | 0 1 ... 8 9 |
SS | 00 01 ... 98 99 | |
SSS | 000 001 ... 998 999 | |
SSSS ... SSSSSSSSS | 000[0..] 001[0..] ... 998[0..] 999[0..] | |
Time Zone | Z | -07:00 -06:00 ... +06:00 +07:00 |
ZZ | -0700 -0600 ... +0600 +0700 | |
Unix Timestamp | X | 1360013296 |
Unix Millisecond Timestamp | x | 1360013296123 |
dir Attribute
The dir
attribute sets the direction of grid's columns and text. ZingGrid supports the dir
attribute inherited from the language or set directly on <zing-grid>
.
dir Demo
Here is a demo that shows one grid with [dir="ltr"]
(text direction left to right) and another with [dir="rtl"]
(text direction right to left):
Sort
If the default language on the page is English, then we use standard sort. If you have any other language defined, you can override sort/filter functionality with the following properties:
- You can turn this off with
<zing-grid sort-intl="disabled">
<zing-grid sort-intl>
and<zg-column sort-intl>
Filter/Search
ZingGrid will properly filter and search non-English characters when defining a language.
[features: internationalization]